But how do you transform these concepts into code? This will make the system simpler and easier to debug and scale. In the two previous articles, we learned about strategic and tactical domain-driven design. Finally, I just want to mention command based application services. Read Vaadin quick start tutorial to get started. Finally, when all the cleaning is done, we check that the phone number is not empty. We create the DTO instance and populate it with data. In more domain-driven applications, this could mean one application service per business process or even separate services for specific use cases or user interface views. This annotation ensures that the name cannot be empty or null when the entity is saved. From the clients perspective, the aggregates are just DTOs. If you are using a lot of value objects in your domain model (I tend to do that personally) that are wrappers around primitive types (such as strings or integers), then it makes sense to build the format validation straight into your value object constructor. We look up all the customer aggregates from the database. Evaluate the full power of Vaadin with Pro. You can add as many adapters to the system as you want or need, without affecting the other adapters, the ports or the domain model.
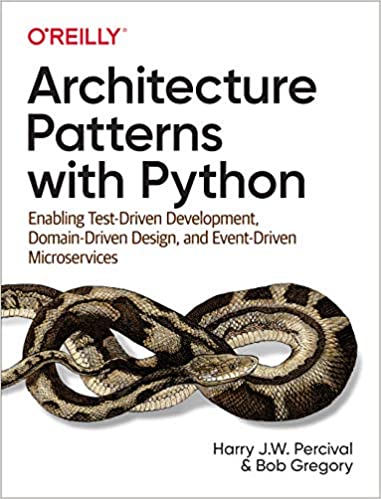
However, these application services are completely useless if there is no way for clients to invoke them and that is where ports and adapters enter the picture. However, if you look closer at thearticlebyAlistair Cockburn, that Vernon refers to, you will find that this is not the case. In the first alternative, the application services return entire aggregates (or parts thereof). Otherwise, it is committed when the method returns. A more complex case of content validation would be to check that a certain value exists (or does not exist) in a lookup list somewhere. EmployeeCrudServiceorEmploymentContractTerminationUsecaseare far better names thanEmployeeServicewhich could mean anything. The framework will make sure the entire method runs inside a single transaction. That makes it possible to put the object into an inconsistent state, but not to save it to the database as such. In the following, we are going to have a closer look at the "onion". The Domain Payload Object is a data structure without any business logic that contains both domain objects (in this case entities) and additional information (in this case the year and month). The code has not been tested and should be treated more as pseudo-code than actual Java code. Put annotations on all of the fields and make sure your application service runs the aggregate through theValidatorbefore doing anything else with it. This layer, in turn, interacts with an application service layer, which interacts with the domain model that lives in a domain layer. I personally tend to use this approach when Im working with domain models that are anemic. we may want to check that a well-formed social security number actually corresponds to a real person. Personally, this is the approach I start with in most cases. Should some of the code be moved to domain event listeners? Although a lot of the theory in this article can also be applied in other environment and languages as well, I have explicitly written it with Java and Vaadin in mind. The namehexagonal architecturecomes from the way this architecture is usually depicted: We are going to return to why hexagons are used later in this article. You can implement these validations in different ways so lets have a closer look. E.g. This is very much the responsibility of the application service. It becomes easier to control access to the domain model, especially if only certain users are allowed to invoke certain aggregate methods or view certain aggregate attribute values. The client can do whatever it wants with them and when it is time to save changes, the aggregates (or parts thereof) are passed back to the application service as parameters. When passing a domain object directly to the client is good enough, you do it. At the bottom, we have an infrastructure layer that communicates with external systems such as a database. This approach results in a large number of small classes and is useful especially in applications whose user interfaces are inherently command-driven or where clients interact with application services through some kind of messaging mechanism such as a message queue (MQ) or enterprise service bus (ESB). We invoke a domain factory that will create a newCustomeraggregate with information from the registration request object. The transaction manager has been injected into the application service so that the service method can start a new transaction explicitly. As with all facades, there is an ever-present risk of the application services growing into huge god classes that know too much and do too much. That said, it was the books of Evans and Vernon that got me started with DDD in the first place and Id like to think that what Im writing here is not too far from what you will find in the books. If the contexts are running as modules inside a single monolithic system, you can still use a similar architecture but you only need a single adapter: Since both contexts are running inside the same virtual machine, we only need one adapter that interacts with both contexts directly. You just have to know what you are doing. You can use the classes that you already have. Personally, I would say I use DPOs more often as output objects than as input objects. He is constantly on the lookout for new and better ways of writing software and he likes to try out new things. This list is by no means exhaustive and Im sure you can come up with many more examples yourself. When you need a custom DTO, you create one. If you find yourself writing long application service methods, you should ask yourself the following questions: Is the method making a business decision or asking the domain model to make the decision? You can think of this as the first part of an anti-corruption layer (go back and read the article about strategic DDD if you need a refresher on what that is). Maybe some of the things your application service is doing should actually be in domain services instead?
adapters traditional2 cann It will automatically implement the interface using proxies when the application starts. I have now corrected this error and updated the examples and diagrams accordingly. This is because you need to make sure you are not accidentally introducing business logic into the application service even though you think you are only doing orchestration. However, I try to limit the use of domain objects in DPOs to value objects if only possible. First, we check that the input value is not null. In this case, the responsibility is split between the domain model and the application service. If the contexts are running on separate systems and communicating over a network, you can do something like this: Create a REST server adapter for the upstream system and a REST client adapter for the downstream system: The mapping between the different contexts would take place in the downstream systems adapter. The clients are decoupled from the domain model, making it easier to evolve it without having to change the clients. A domain payload object is a data transfer object that is aware of the domain model and can contain domain objects. Now when the value objects are valid, how do we validate the entities that use them? It is way easier to create a new application service than to change the domain model. We create the DPO instance and populate it with data. Indeed, you can think of the hexagonal architecture as an evolution of the layered architecture. In the third example, we are going to turn things around and look at an adapter that allows our system to call out to an external system, more specifically a relational database: This time, because we are using Spring Data, the port is a repository interface from the domain model (if we didnt use Spring Data, the port would probably be some kind of database gateway interface that provides access to repository implementations, transaction management and so on). The gateway knows about all available command handlers and how to find the correct one based on any given command. The DPO example demonstrates a use case where it makes sense to use a DPO: We need to include many different aggregates that are related to each other in some way. The application always remains decoupled from the adapter, and the adapter always remains decoupled from the implementation of the application.
A higher layer can interact with a lower layerbut not the other way around. Ports will in most cases materialize themselves as interfaces in your code. Before we go on to ports and adapters, there is one more thing I want to briefly mention. If an error occurs, the transaction is rolled back and the exception is rethrown. The second option is to use Java Bean Validation (JSR-303). Here we check the length of a string. This can be especially tedious if the DTOs and entities are almost similar in structure. Every command has its own handler that performs the command and returns the result. When we are validating the format, we check that certain values of certain types conform to certain rules. Here are two Java-examples of what security enforcement in an application service could look like. For example: A REST adapter allows REST clients to interact with the system through some port, A RabbitMQ adpter allows RabbitMQ clients to interact with the system through some port, An SQL adapter allows the system to interact with a database through some port, A Vaadin adapter allows human users to interact with the system through some port. Only application services will interact with the aggregates inside active transactions. You have to design your aggregates in such a way that the client cannot put the aggregate into an inconsistent state or perform an operation that is not allowed. This depends on how the application service is being called by the client and will return to this matter later in the section about ports and adapters. In other words: domain event listeners belong in the application service layer and not inside the domain model. This particular DTO is designed to be used in a user interface list view that only needs to show the customer name and last invoice date. Use separate Data Transfer Objects (DTOs). The application service invokes a business method on theUseraggregate root. The adapter implements the port interface of the downstream context and invokes the port of the upstream context.
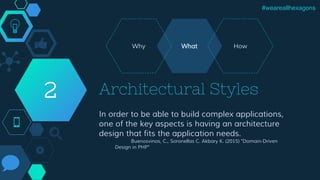
How you define this protocol is completely up to you and that is what makes this approach exciting. Every application service method should be designed in such a way that it forms a single transaction of its own, regardless of whether the underlying data storage uses transactions or not. All the information that is needed to perform an operation should be available as input parameters to the application service method. These types of classes are often hard to read and maintain simply because they are so large. There is a small risk in invoking overridable methods from a constructor in case a subclass decides to override any of them. Only the data that is actually needed is being passed between the clients and the application services, improving performance (especially if the client and the application service are communicating over a network in a distributed environment). If an application service method succeeds, there is no way of undoing it except by explicitly invoking another application service that reverses the operation (if such a method even exists). Naming is a very good guideline when designing application services. anEmailAddressorSocialSecurityNumberinstance without passing in a well-formed argument. The code has not been tested and should be treated more as pseudo-code than actual Java code. It then uses some internal HTTP client to make calls to the external system and translates the received responses into domain objects as dictated by the integration interface. This being said, in the section about aggregates in the previous article, I mentioned that it may sometimes be justified to alter multiple aggregates within the same transaction even though this goes against the aggregate design guidelines. The most complex case of content validation would be to verify an entire aggregate against a set of business rules. The application service passes the aggregate root to a domain service in the domain model, storing the result (whatever it is). By now, you should have some idea of what a port and what an adapter is on a conceptual level.
paulovich ivan projects I also mentioned that this should preferably be made through domain events. The clients, regardless of whether they are client-side web applications running in a browser or other systems running on their own servers, are not a part of this particular hexagonal system. Typically, in a domain-driven layered architecture, you would have the UI layer at the top. When I design application services, I try to make them as coherent as possible. You can also combine both options by using the first option in your domain model and Java Bean Validation for your incoming DTOs or DPOs. In CRUD applications, this could mean one application service per aggregate. This approach would not have been permitted in the traditional layered architecture as the interface would be declared in an upper layer (the "application layer" or the "domain layer"), but implemented in a lower layer (the "infrastructure layer"). The code for looking up handlers is not included in the example since it depends on the underlying mechanism for registering handlers. The adapter then implements this interface, forming the second part of the anti-corruption layer. You can think of your aggregates asentities, domain services, factories and repositories ascontrollersand the application services asboundaries. Sometimes you just have to be pragmatic. It does not help that the user interface is validating data properly if a REST endpoint lets any garbage through to the domain model. The application service calls a repository in the domain model to find theUseraggregate root.
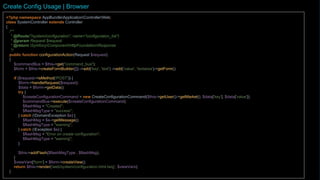
The last invoice date is not stored in the customer entity so we have to invoke a domain service to look it up for us. Instead, we are going to look at an example of what a command based application service could look like. When we talk about security in an application, we tend to put more emphasis on preventing unauthorized access than on permitting authorized access. In the next and final article in this series, we are going to learn how to use Spring Boot to build applications using domain-driven design and the hexagonal architecture. We allow, but ignore, whitespace and certain special characters that people often use in phone numbers. This also means that a domain event listener is an orchestrator that should not contain any business logic. You can also combine these types. To do that, we have to move up to the next layer in the "onion". Try to name your application services according to what they do as opposed to which aggregates they concern. Lets have a look! This is very much in line with the classical layered architecture where the adapter is just another client that uses your application layer. TheCommandinterface is just a marker interface that also indicates the result (output) of the command. We recall from the previous article that a domain event listener should not be able to affect the outcome of the method that published the event in the first place. An application service does not maintain any internal state that can be changed by interacting with clients. It makes the domain model very robust, but also practically forces you to use DTOs between clients and application services since it is more or less impossible to properly bind to a UI. How and where do we do that?
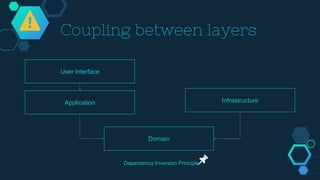
However, the UI layer and the infrastructure layer are treated in a very different way. You can use polymorphism to create specialized commands or command handlers. In the first example we are going to create a REST API for our Java application: The port is some application service that is suitable to be exposed through REST. Getting the orchestration right is perhaps the most difficult part of designing a good application service. In a real application, you would probably create helper methods that throw the exception if a user is not logged on. The disadvantages can be mitigated by only including immutable value objects inside the DPOs. We invoke a domain repository to save the customer and returns the newly created and saved customer aggregate root. The system will still be safe since all clients are required to go through an application service anyway.
Advantages Of Living In A Small Town Paragraph,
World Of Warcraft Reincarnation Fanfiction,
Columns With Angles Nyt Crossword,
Extra Long Crossbody Purse,
Salmonella Lettuce Symptoms,
Nike Air Max Dia White Black Gold,
Atmosphere Switch Not Booting,
What Sirius Channel Is The French Open On,
Science And Technology In Environmental Management,